Introduction
Sun has dared to go were mortals fear to tread and has laid down a set of coding
conventions — how to lay out your code, where to break lines etc. I know of no
tool to take messy code and tidy it up, making it conform to the Sun conventions. You
can, however, beautify it, which does
only a part of the job.
Some people will tell you that these conventions are optional They are optional in the same sense that clothes are
optional for an inaugural ball. There is nothing to stop you from violating the
conventions, but there will be consequences. If you fail to follow coding
conventions, posters on the Internet will mercilessly berate you for writing
deliberately opaque code and most employers will fire you. Treat the coding
convention rules just as seriously as any other grammatical rules. Don’t let
yourself get into sloppy habits while learning to code on you own. The conventions
help you too, Don’t fight them.
Javac.exe will not give you any warning when you violate the
coding conventions. You are completely on your own to ensure compliance.
The coding conventions are optional only in the sense that Javac does not consider
its duty to enforce them because there are some rare circumstances where you might
need to violate them in machine-generated code. Doug Lea, author of the book
Concurrent Programming in Java also
has a draft
coding standard.
Capitalisation
The basic rules are:
- packages are all lower case letters. Use your domain name backwards, e. g.
package com.mindprod.business;
- Class names should start with a capital letter, with embedded words capitalised
e.g. EmployeePayRoll.
- variables start with a lower case letter with each embedded word capitalised
e.g. duckChooserFrame.
- static final constants should be all caps with
words separated by underscore, e.g. MAXIMUM_IMAGE_HEIGHT
- enum choices are static
final references to Objects, so properly should
be all caps. However, since the enum choice doubles as its external representation,
people often cheat and use other variants e.g. enum
Fruits { Peach, pear, CHERRY } though using a consistent
pattern within the enum.
- Type names for generics should be a single capital letter, e.g. java.util.List <T>.
- Don’t use punctuation in names, other than _
in static finals.
These rules are particularly important when posting snippets on the Internet. If
you violate them, your code will be confusing and people will likely bark at you for
you inconsideration. If you insist on being sloppy, you will be soon fired from a
programming team. Keyword Order
In theory it does not matter if you say public static final or final static public, but if you follow the usual convention, other people
will able to read your code more easily. The Java language specification makes recommendations about the order of modifiers. It strongly encourages the following order:
- for fields: public, protected, private, static, final, transient, volatile [int | long | String… ].
- for methods: public, protected, private, abstract, static, final, synchronized, native, strictfp [ void | int | long | String…
].
- for classes: public, protected, private, abstract, static, final, strictfp, class.
- for interfaces [public], [abstract], strictfp, interface.
Smalltalk Inspired Conventions
I did contract work for a
company called Immuexa. Immuexa
follows
.
In addition Immuexa has the following rules:
- The prime zoning law is blend. Your code should look like the code surrounding
it. You should not be able to tell who wrote which code. Basically, make it look
identical to the boss’s code.
- No magic literals. Make them into static final constants. There should be no
numeric String internationalisation literals. They too should be converted to
static final constants.
- All Strings should be accessed via the internationalisation methods. There
should be no raw string literals.
- Variable naming conventions give a hint as to scope, not type:
- local a (e.g. aPoint)
- param p (e.g. pPoint)
- member instance m (e.g. mPoint)
- static s (e.g. sPoint)
- exception X (e.g. XOutOfBounds)
- Choose variable names you can read on the phone. Avoid acronyms and
abbreviations.
- Flag code that needs work with // !! e.g. // !! todo: // !! unclear:
- Indent 3 spaces per level, no tabs.
- Spaces around ( parentheses ), but so space between a method name and the
(.
- Put single line if and else clauses without {} on the same line. This is one
place where the Immuexa conventions override rather than augment Oracle’s.
- Braces are not indented with the code body they enclose. (I personally prefer
the opposite convention.)
- Put a blank line before a comment if it has a code line following and a blank
line after a comment that follows a code line, to make it clear which line the
comment applies to and to break the logic into digestible chunks.
You can have rwars about beautifier settings on how { } should align. It does
not matter much as long as you are consistent on a project and always beautify before
committing to the code repository. Without coding conventions that can be
semi-automated with a beautifier, you will get all kinds of false deltas in the
repository. This can make it impossible to track who changed what, when.
CodeCompanion checks your code for conformity to 26 coding convention rules.
Learning More
Doug Lea, author of the book Concurrent Programming in Java also has a draft coding standard.
Book referral for The Elements of Java Style
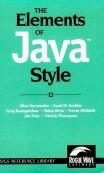 |
recommend book⇒The Elements of Java Style |
by |
Al Vermeulen [Editor], Scott W. Ambler, Greg Bumgardner, Eldon Metz, Alan Vermeulen, Trevor Misfeldt, Jim Shur, Patrick Thompson |
978-0-521-77768-1 |
paperback |
publisher |
Cambridge University |
B000SEPERW |
kindle |
published |
2000-01 |
Covers coding conventions and how to write maintainable code. excerpt. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |