an application designed to run on a server in the womb of a permanently resident
CGI (Common Gateway Interface) mother program written in Java that provides services for it, much the way an Applet
runs in the womb of a Web browser.
Servlet Advantages
Servlets have the following advantages
over CGI.
- A Servlet does not run as a separate process. This removes the overhead of
creating a new process for each request.
- A Servlet stays in memory between requests. A CGI
program (and its runtime support) needs to be loaded and started for each
CGI
request.
- There needs to be only one copy of the servlet in RAM (Random Access Memory)
at a time. Using threads, it can service several requests simultaneously. With
CGI there
is one copy of the code for each transaction in process.
- The servlet sandbox can watch over the servlets to make sure they behave
themselves. In traditional CGI
programming, a coding error can bring down the entire system.
Servlet Extensions
It gets tedious writing Servlets that embed reams of
HTML (Hypertext Markup Language) inside Java strings, since
your outputs are complete HTML pages. So people have created scores of rinky dink
ways of embedding Java code in HTML
that gets compiled into the equivalent Java Servlet code dynamically. I find them all
disgustingly amateurish. You might have a look at some of the choices of framework.
There are various ways of writing server code that build on top of the basic
Servlet classes. JSP (Java Server Pages) and Freemarker let you embed bits of Java code and
other scripting inside your HTML pages. These are parsed and converted to Java Servlet
programs that dynamically generate HTML
with variable fields. These are automatically recompiled as needed.
With JSP all you have to do is drop the *.jsp files in a magic directory and away you go. With Servlets you must
put the *.class files or *.war
files in a magic directory and register the servlets in a web.xml file that maps application name to servlet class among other
things.
Writing Servlets in XML (extensible Markup Language)
In the following
web.app example, the user types http://localhost:8080/hello. That is mapped to a servlet named
hello-world. That servlet is implemented by the Java Servlet
test.HelloWorld.class.
<web-app>
<servlet-mapping url-pattern='/hello'
servlet-name='hello-world'/>
<servlet servlet-name='hello-world'
servlet-class='test.HelloWorld'/>
<init-param greeting='Hello, world'/>
</web-app>
Under the Hood
The big puzzle to the
novice is where are the jars for the Servlet classes?
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.ServletException;
Sun does not provide the jars. You get them from your servlet womb vendor —
his particular implementation of the interfaces. If you use Caucho Resin, it will
automatically use its version of the classes and automatically recompile your java
source as-needed. You have to read the womb docs. Often they make you copy the entire
set of system jars to your own directory for use.
javax.servlet.Filter is an interface to allow a
transaction to be processed in an assembly line. Each Filter does some of the work and passes on the input or output to
the next filter in the chain and decides on who is next.
Getting Started
Getting your first HelloServletWorld working can be quite a challenge.
Debugging Tips
Learning More
javax.servlet.http.HttpServlet docs : available:
javax.servlet.http package docs : available:
javax.servlet package docs : available:
Books
Book referral for Head First Servlets and JSP: Passing the SCJP Oracle Certified Web Component Developer Exam
 |
recommend book⇒Head First Servlets and JSP: Passing the SCJP Oracle Certified Web Component Developer Exam |
by |
Bryan Basham, Kathy Sierra, Bert Bates |
978-0-596-51668-0 |
paperback |
publisher |
O’Reilly  |
978-1-4493-6086-3 |
eBook |
published |
2008-08-07 |
B009Z45JAI |
kindle |
A very complete book, partly because it aims to prepare you for the Oracle exam. It is also a difficult book. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Core Servlets and Javaserver Pages: Advanced Technologies, Vol. 2, second edition
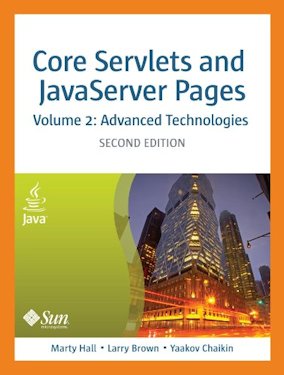 |
recommend book⇒Core Servlets and Javaserver Pages: Advanced Technologies, Vol. 2, second edition |
by |
Marty Hall, Larry Brown, Yaakov Chaikin |
978-0-13-148260-9 |
paperback |
publisher |
Prentice Hall |
978-0-13-271568-3 |
eBook |
published |
2007-12-01 |
B004YWAZFA |
kindle |
Complete text of the book available on line in pdf format. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Developing Enterprise Java Applications with EE and UML
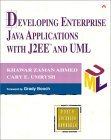 |
recommend book⇒Developing Enterprise Java Applications with EE and UML |
by |
Khawar Zaman Ahmed, Cary E. Umrysh |
978-0-201-73829-2 |
paperback |
publisher |
Addison-Wesley |
published |
2001-10-27 |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Web Development with JavaServer Pages
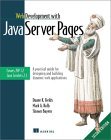 |
recommend book⇒Web Development with JavaServer Pages |
by |
Duane K. Fields, Mark A. Kolb, Shawn Bayern |
978-1-930110-12-0 |
paperback |
publisher |
Manning |
published |
2001-09-15 |
A very thorough treatment. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Inside Servlets: Server-Side Programming for the Java(TM) Platform, second edition
 |
recommend book⇒Inside Servlets: Server-Side Programming for the Java(TM) Platform, second edition |
by |
Dustin R. Callaway, Danny Coward |
978-0-201-70906-3 |
paperback |
publisher |
Addison-Wesley |
published |
2001-05-14 |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Java Servlet Programming, second edition
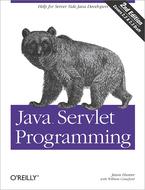 |
recommend book⇒Java Servlet Programming, second edition |
by |
Jason Hunter, William Crawford |
978-0-596-00040-0 |
paperback |
publisher |
O’Reilly  |
978-1-4493-9067-9 |
eBook |
published |
2001-03-01 |
B0043M4Z8E |
kindle |
highly recommended. Covers basics of JSP too. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |