JSP (Java Server Pages)
is a language similar to Microsoft’s ASP (Active Server Page)
for creating dynamic web pages. Sun has created a language specification. With
JSP,
a single source textfile is created containing both HTML (Hypertext Markup Language)
(or XML (extensible Markup Language)) tags and Java-like scriptlets. The JSP-aware web
server composes, compiles and runs a Servlet from the
source text. For increased efficiency, the JSP-aware server will typically cache the
compiled Servlets. The compiled form are perfectly
ordinary Servlets and thus can be mixed in with ordinary
Servlets.
Part of the magic of JSP is that you can replace JSP
source code on the fly while the server is active. The next time a request
comes for that JSP code, will be automatically recompiled. You
don’t have to shut down the server ever time you change the text or
JSP markup of a page.
This works because of clever use of classloaders to see that obsolete classes are
garbage collected. Normally, you use this feature only for testing. You normally
don’t update your code while a production server is running.
Multiple Languages
To master JSP,
you need to master several languages:
Hello.jsp
Here is a the JSP equivalent of the HelloWorld program.
See servlet for instructions on how to
install and run it. The Servlet womb will automatically convert the *.jsp to *.java and compile it to a
Note how it looks like ordinary HTML
with dynamically generated variable content Java code enclosed in <%=… %>. Inside those delimiters is a Java expression,
called a scriptlet, that when evaluated will produce a
String to insert at that point in the
HTML file.
Have a look at how various JSP
files expand into Java source code to generate and glue the various bits of text
together. In addition there are other things called tags
you can embed with many different syntaxes. Unfortunately, the documentation on how
to use them reads like the ramblings of someone with ADD. They keep wandering off on
tangents before they have explained the core functionality. Further, they
scrupulously avoid using examples to explain the abstract gibberish. This is ad hoc
language creation at its worst.
By default the Servlet generated is thread-safe so the
same code can process several requests simultaneously. Alternatively, you can declare
your code with <%@ page isThreadSafe=false %> so that your Servlet will
only be used to process one message at a time.
Note that the <%=…%> delimiters used by JSP
are not legitimate HTML. They must be expanded and removed before feeding the
page to a browser. (In contrast, static macro markup are legitimate
HTML
even before expansion and are not removed on expansion.)
There are many different sorts of beast you embed in your
HTML. Among
them are the following tags:
- <%=… %>
- <jsp:… />
- <%@ page… %>
- <%@ include… %>
- <c:forEach var=row
items=${rs.rows}>
- <tr class=${(stat.index & 1) == 0
? evenRow : oddRow}>
In that last example, the <tr is just an
ordinary table row command, nothing to do with JSP.
The JSP part is the
${…}
A *.jsp file generates an entire page
of HTML. Tags
generate just a snippet of boilerplate to include in a page. They can have both
mandatory and optional parameters, but I have not yet learned how to handle a
variable number of parameters. Tags are like macros in other languages. You implement
the tag by writing a class that extends
javax.servlet.jsp.tagext.TagSupport.
The Javadoc is inscrutable. You will have to figure out what it means from examples
and web tutorials. You also have to write a *.tld
TLD (Top Level Domain) file, containing XML
to describe the names of the tags, the parameters and the name of the class to
process the tag.
There are a number of general purpose tag libraries (taglibs) available on the net that you can access simply by giving the
URL (Uniform Resource Locator) of their *.tld files.
Here
You can have one-liner bodyless tags or begin-end tags enclosing a body. The body
is like a large parameter, often of text to format. The syntax is borrowed from
XML.
You must enclose parameters in either " or
'. If the attribute value itself contains "s, you must enclose the parameter value in '. If the attribute value itself contains 's, you must enclose it in "…". What do you do if a string contains both
" and '? You must use the
entity " for embedded " and surround the string in "s, e.
g.:
<mytag:album title="Sargeant
Pepper’s Lonely Hearts Club Band">
<mytag:album title='The Wall'>
<mytag:album title="Peter’s
"Weird Songs"">
Two Types of Include
<jsp:include> is a dynamic, runtime/request time include. It
invokes another class to produce a page and includes that result embedded in the page
output.
<%@ include> is a macro-style, inline,
translation/compile time include that inserts some JSP
/ HTML source
code into the current JSP
program.
Potential of JSP
I don’t
think websites have even begun to exploit the power of JSP
yet. Here are some things you potentially could do with it:
- Serve different style sheets to lets your viewers choose colour schemes/skins
and type size.
- Adjust the layouts to the size of the browser screen, anywhere from a cellphone
to a dual airport sized plasma display.
- Prepare a version of the website for download and offline viewing with the
Replicator or for local HTML
validation.
- Depending on speed of the connection, select resolution/size of images.
- Display prices in local currency units.
- Display dates in local date format.
- Automatically applying the correct width and heights on all image
references.
- Move ads around, change colours, change them, alternate them with
PSA (Public Service Advertisement)
ads, quotations and other messages, so that people don’t tune them out
completely.
- Customise Google links to the national server site.
- Show temperatures is F or C depending on country.
- Display units of measure in imperial or metric (or both) depending on
country.
Alternatives To JSP
There are two
basic alternatives to JSP :
- Ones that also rely 100% on server side computing,
such as Velocity, Tea and Freemarker and other frameworks. There are scores of lemmings all going for the
fundamentally flawed, but easy to implement, 100% server
side solution. You can see a list of them under servlet wombs.
- Ones that use a combination of server side and client side computing such as
Flash is about the only mainstream
alternative, (unfortunately non-Java), for the combined solution. I have written up
a JSP Replacer student
project for an all-Java server-controlled client-side validation environment.
Gotchas
- Your UEL (Unified Expression Language) markup will be ignored unless you place a page
directive at the top of the page like this:
<%@ page isELIgnored=false
%>
Advantages
- The big advantage is in maintaining boilerplate. You change it in only one
place and it is automatically propagated. This is true of static macros as
well.
- There is no extra overhead for having every page different, e.g. with a random
quote of the day.
- You can collect information as well as disseminate it.
- When a user is downloading a page, that does not stop you from uploading a new
version of it simultaneously.
- There is less uploading traffic. You don’t have to upload all the
expanded boiler plate. You don’t have to reupload your
HTML files
simply because the expanded boilerplate of static macros (or hand maintenance) has
changed.
- You can dynamically change what you send out based on facts you know about the
user, such as time zone, country, language.
- You can dynamically change what you send out based on time and date, e.g.
noting anniversaries of births, deaths or marriages. You can do this with static
macros, but it means uploading every time the display changes.
Disadvantages
I am not the only one
who thinks JSP is not the greatest thing since sliced bread. Check
out these essays:
- Jason
Hunter’s essay
- Reaction to Jason
Hunter’s essay
JSP
is like a 400 year old house where each generation
that lived in it tacked on an extra room. There is no overall plan, just a
hodge-podge of architectural styles kludged together. The syntax is, to be
kind, appalling.
~ Roedy (1948-02-04 age:70)
- Muddling your HTML and procedural code together means you expose your
code to the web designers who know only HTML.
They can easily damage it because they don’t understand the markup and
scriptlets.
- Muddying your HTML and procedural code dilutes your code with reams of
irrelevant text.
- You must be much more careful with JSP
with testing before you post — one slip and you take down your entire site
until you fix the problem. With static content, or static macros, you usually muck
up at most one page at a time.
- The tag syntax for doing things like conditionals or loops is clumsy compared
with the way you do it in plain Java.
- It may be unwise to write a lot of code in some non-standard Mickey Mouse
markup language. What happens when the world inevitably abandons it for something
better?
- Your entire website must fit on the heap, double its usual size because of
16-bit characters. The hardware requirements to serve
such a website are obviously bigger than to serve the same information statically.
In addition, the javac.exe compiler must be in
RAM (Random Access Memory) all the time.
- You need to take your server down periodically, especially if you have made a
number of changes.
- Your server is more delicate than a simple HTML
server, more apt to crash. Your coding bugs can take it down. It is more
susceptible to security holes and others exploiting your code to turn against
you.
- URLs (Uniform Resource Locators)
don’t have the familiar *.html extension. When you
flip from a static HTML to JSP,
all the bookmarks people have into your site will stop working unless you
create redirects for all of your old URLs.
Outstanding Questions
- Do you have to rename all links on the website to be *.jsp or can *.html be bulk re-routed?
- Con you invent your own tags with your own internal grammar?
- To add a new JSP page, what do you have to do?
Books
Book referral for Head First Servlets and JSP: Passing the SCJP Oracle Certified Web Component Developer Exam
 |
recommend book⇒Head First Servlets and JSP: Passing the SCJP Oracle Certified Web Component Developer Exam |
by |
Bryan Basham, Kathy Sierra, Bert Bates |
978-0-596-51668-0 |
paperback |
publisher |
O’Reilly  |
978-1-4493-6086-3 |
eBook |
published |
2008-08-07 |
B009Z45JAI |
kindle |
A very complete book, partly because it aims to prepare you for the Oracle exam. It is also a difficult book. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Murach’s Java Servlets and JSP, second edition
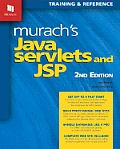 |
recommend book⇒Murach’s Java Servlets and JSP, second edition |
by |
Joel Murach and Andrea Steelman |
978-1-890774-44-8 |
paperback |
publisher |
Mike Murach |
published |
2008-01-21 |
Presumes Tomcat and Netbeans. That is a disadvantage if you use other tools but a boon if you use them, since so many problems are about the details of configuring and implementation, not the code itself. book website |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Core Servlets and Javaserver Pages: Advanced Technologies, Vol. 2, second edition
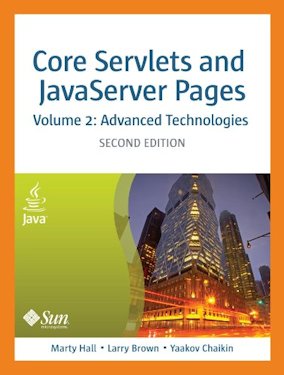 |
recommend book⇒Core Servlets and Javaserver Pages: Advanced Technologies, Vol. 2, second edition |
by |
Marty Hall, Larry Brown, Yaakov Chaikin |
978-0-13-148260-9 |
paperback |
publisher |
Prentice Hall |
978-0-13-271568-3 |
eBook |
published |
2007-12-01 |
B004YWAZFA |
kindle |
Complete text of the book available on line in pdf format. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Java 2 Bible
 |
recommend book⇒Java 2 Bible |
by |
Justin Couch and Daniel H. Steinberg |
978-0-7645-0882-0 |
paperback |
publisher |
Wiley |
published |
2002-04-01 |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for More Servlets and Java Server Pages
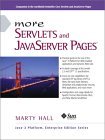 |
recommend book⇒More Servlets and Java Server Pages |
by |
Marty Hall |
978-0-13-067614-6 |
paperback |
publisher |
Pearson Education |
published |
2001-12-26 |
Complete text of the book available on line in pdf format. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Web Development with JavaServer Pages
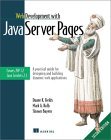 |
recommend book⇒Web Development with JavaServer Pages |
by |
Duane K. Fields, Mark A. Kolb, Shawn Bayern |
978-1-930110-12-0 |
paperback |
publisher |
Manning |
published |
2001-09-15 |
A very thorough treatment. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Core Web Programming, second edition
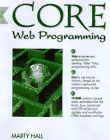 |
recommend book⇒Core Web Programming, second edition |
by |
Marty Hall and Gary Cornell |
978-0-13-089793-0 |
paperback |
publisher |
Prentice Hall |
978-0-613-92274-6 |
hardcover |
published |
2001-06-03 |
1250 pages. Also has some simple RMI examples. This is a great doorstop of a book. It has a few chapters on client-server programming in Java and a section of that is on CGI. I have looked at hundreds of Java books and found nothing that deals in depth with client side Java talking to CGI, except Marty’s book. It is really very simple and he does an excellent job of explaining it. Marty has posted all the source code examples from the book for anyone to use. These contain updates and errata fixes you don’t get on the CD-ROM that comes with the book. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Java Servlet Programming, second edition
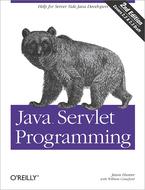 |
recommend book⇒Java Servlet Programming, second edition |
by |
Jason Hunter, William Crawford |
978-0-596-00040-0 |
paperback |
publisher |
O’Reilly  |
978-1-4493-9067-9 |
eBook |
published |
2001-03-01 |
B0043M4Z8E |
kindle |
highly recommended. Covers basics of JSP too. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Book referral for Pure JSP: Java Server Pages
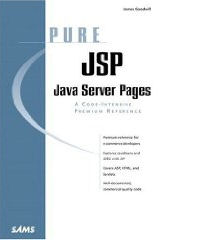 |
recommend book⇒Pure JSP: Java Server Pages |
by |
James Goodwill |
978-0-672-31902-0 |
paperback |
publisher |
Sams |
published |
2000-06-08 |
I would not recommend this book. It seems as it were constructed by copy/paste from the spec. It tries to explain things abstractly without even explaining the conventions of his BNF notation. It needs to be rewritten replacing the BNF with concrete, realistic examples. Reading this book is likely listening to a politician trying to avoid a question. It does have examples, but beats around the bush needlessly before getting to the point. Does not cover tags or UEL. |
|
Greyed out stores probably do not have the item in stock. Try looking for it with a bookfinder. |
Learning More